Add to home screen feature gotchas
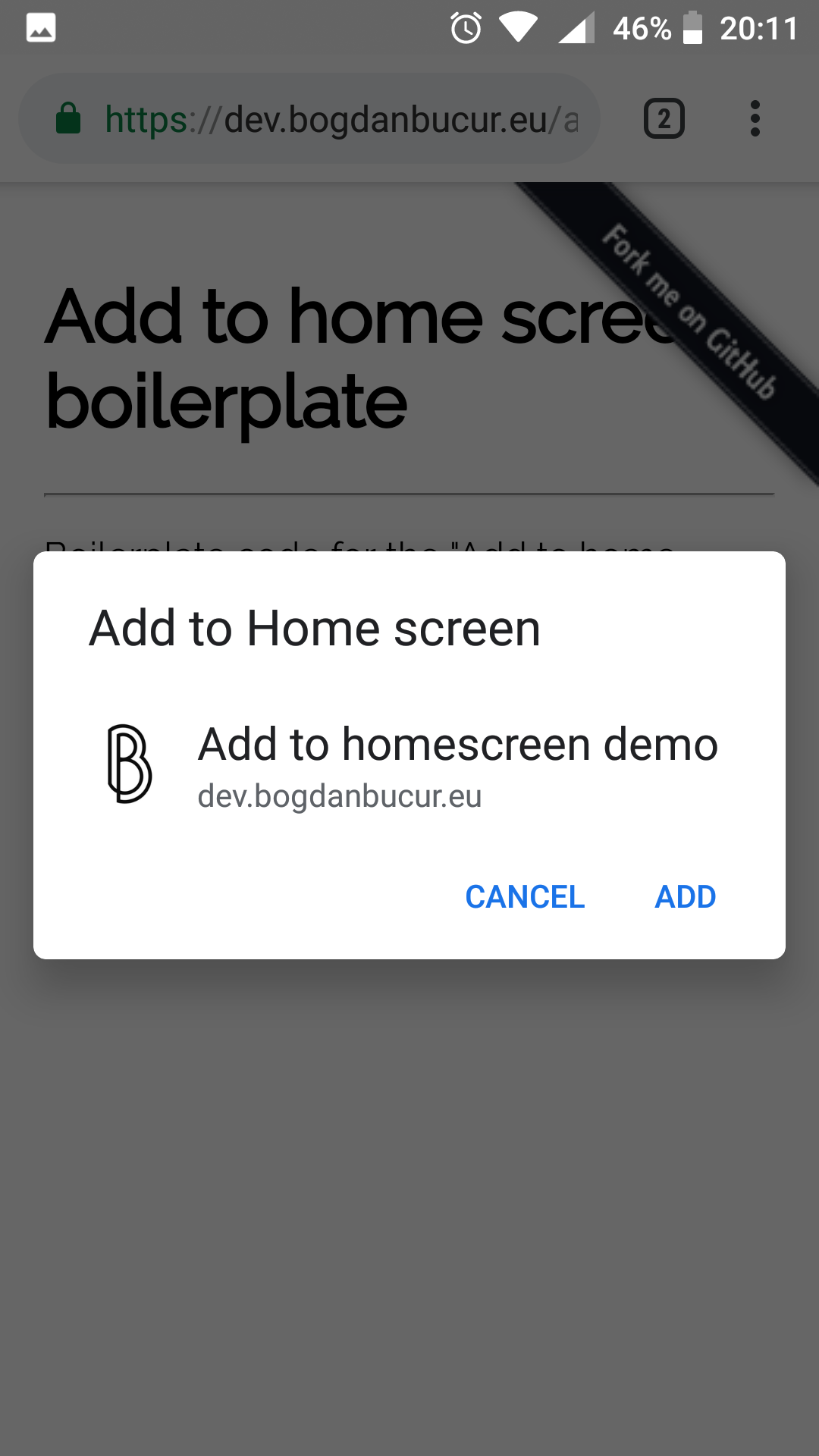
One of the basic features of a modern progressive web app, the add to home screen option looks like it can be taken care of in a couple of minutes, or so I presumed. Little did I know about what awaited me, and the weakly documented gotchas that I had to face made the whole ordeal take well over an hour.
In all fairness, Google's "Add to Home Screen" tutorial gets you 95% there. It's those last percents that get you.
Step 1: add an app manifest
As easy as adding a simple link tab inside the <head>
:
<link rel="manifest" href="manifest.json">
it gets you with the start_url
property.
Things are straightforward if you want to set it up for your website but it gets tricky when you want to create a short-cut for a relative path. Case in point, my own demo page at dev.bogdanbucur.eu/add-to-homescreen/.
In order to have a shortcut towards /add-to-homescreen
I had to add a point to the start_url: start_url: './index.html'
(Gotcha!).
Simply using start_url: 'index.html'
would not have used the path relative to the manifest.json file's location, but it would have went straight to dev.bogdanbucur.eu/index.html.
Step 2: add the service worker
Simply add a <script>
tag inside the <head>
with the following content
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('service-worker.js')
.then(function(reg){
console.log('service worker registered', reg);
}).catch(function(err) {
console.log("service worker not registered. Error: ", err)
});
}
If you're wondering what Has registered a service worker with a fetch event handler means, it's easy (but not well explained).
Just make sure the service-worker.js file contains this code (Gotcha!)
this.addEventListener('fetch', function(e){
});
Save it as service-worker.js inside the root folder (dev.bogdanbucur.eu/add-to-homescreen/service-worker.js
in my case) and you're ready to go. Just don't leave it empty, as some other tutorials suggest.
Step 3: handle the "Add to homescreen" dialog
While Google tutorials tells you you can defer the beforeinstallprompt
event, in order to display a mesage of your own to the user, in practice it is ignored by the browser, at least when I tried it.
It makes some sense when you think about it. Even though there are legitimate use cases for this features, there are even more cases when it is used for spammy purposes. The last thing the web needs are another set of flashy, disturbing, animated popups, begging you to add the site to your home screen.
Therefore, you should stick to the basics with the code below, useful in case you need to save stats or user preference data regarding the installs.
window.addEventListener('beforeinstallprompt', function (e) {
// Prevent Chrome 67 and earlier from automatically showing the prompt
e.preventDefault();
// Show the prompt now
e.prompt();
// Wait for the user to respond to the prompt
e.userChoice
.then(function (choiceResult) {
if (choiceResult.outcome === 'accepted') {
console.log('added to homescreen');
} else {
console.log('not added to homescreen');
}
});
});
What now?
All the code is in place and you're wondering what to do next. For me, the next step was to make a quick test. And how do you do it without opening your phone and tablet? With Chrome's developer tools Audit section.
Simply select the basic options, like in the screenshot below: Mobile, Progressive Web App and No Throttling (only Progressive Web App is mandatory, the rest are simply chosen to make the test faster).
Click "Run" and wait for the results.
If there are some issues with the "Add to home screen" implementation, the Audit will point it out, as well as give you tips on how to solve it. But there's a huge Gotcha! there, one that almost made me lose my mind.
Sometimes the test returns false negatives, a known issue of the tool. So if the url looks ok to you, just ignore that warning and go ahead with the test on the actual device.
Assuming you had some interraction with the website, you will see an install banner in the bottom of the page, like in the screenshot below.
Click on it and you will get a prompt to do the actuall "add to home screen"-ing part of the process, the one that triggers the event listenere from step 3.
Careful while testing, this features does not work in incognito mode (Gotcha!), something I learned the hard way.
If anyone else need this: Boilerplate code for the mobile "Add to homescreen" feature