What do you do when you need a large number of different images with various sizes? You make a Python script
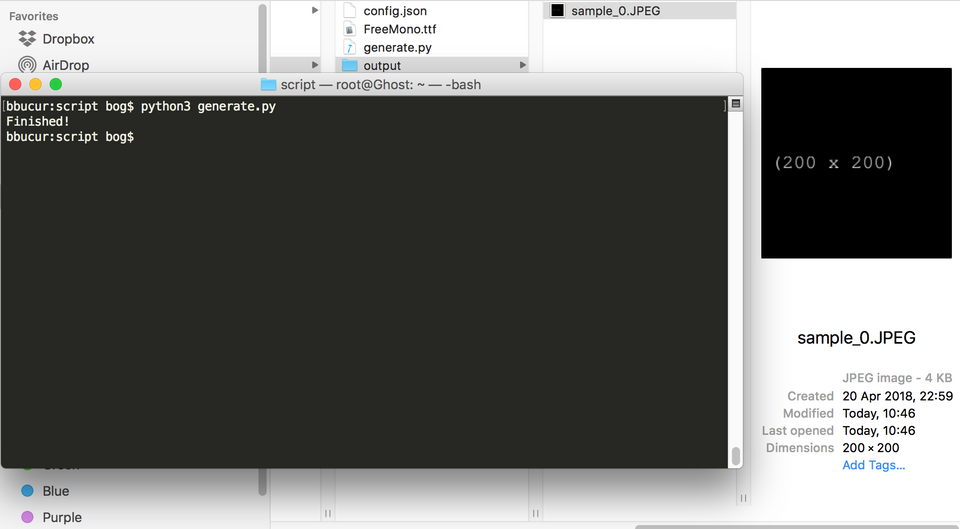
Since the advent infinite scrolling, one of the most common problems in development is loading a large number of images without crashing the browser. Combine it with the grid display a la Pinterest or Masonry and you get a nice and interesting problem that I believe any web developer should solve at least once.
Having ran into this challenge many times in the past, in various forms, the biggest problem was not on the code side but it was more of a... logistics issue.
I was in need of a large number of images, maybe as high as a few thousands. They had to be different, since repeating the same image is catched by the cache and they sometimes had to have different ratios / sizes as well (for properly testing a Pinterest grid display).
While by no means a true Python programmer, it's always my go-to scripting language when I need something done good and quickly.
With Pillow doing all the heavy lifting, I finally made the switch to Python 3 and made myself an image generating script to solve my problem.
The code below represents a simple method of drawing a 100x200 pixels black image, with the text "Hello World" written in white, 20 pixels font size, drawn at the 10x10 top left corner of the image and the saved as a JPEG with max quality.
from PIL import Image, ImageDraw, ImageFont
# drawing a 100x200 pixels image with a black background
image_size = (100, 200)
background_color = '#000000'
img = Image.new('RGB', background_size, background_color)
# drawing a white text, 20 pixels font size, at the 10x10 coordinates
draw_context = ImageDraw.Draw(img)
font_size = 20
text_font = ImageFont.truetype('my_font_file.ttf', font_size)
text_color = '#FFFFFF'
text_position = (10, 10)
draw_context.text(text_position, 'Hello world', font=text_font, fill=text_color)
#saving the image as a max quality JPEG
image_format = 'JPEG'
img.save('image.jpg', , quality=100)
As you can see, it's pretty straightfoward, with a simple python generate.py
command and configurable via a local config.json
file, which can also be created online with the Py Image Generator configurator, for those rare people who might not feel comfortable with the json structure, you can easily create how many images you need, with different sizes and colors and following a particular naming convention.
You can check out the code on Github: Py Image Generator or on the Py Image Generator project page.